¿Qué es Text?
El componente Text
será usado para mostrar texto en la aplicación. Es un componente equivalente a UILabel
de UIKit
.
Si os interesa, podéis consultar la documentación oficial aquí.
La creación de este componente se realiza de la siguiente forma:
Text("Hello, World!")
Modificadores comunes para Text
A parte de los modificadores que se explican a continuación, el componente Text
comparte los mismos métodos de personalización que el componente View
y pueden ser consultados en este enlace.
lineLimit
Indica el número de líneas máximo que puede tener el texto. Por defecto tiene el valor nil
, que indica un número de líneas ilimitadas.
Text("This is a long text with line limit set to 2. I need more text for ensure is a real multiline text, so I repeat all to say before. `This is a long text with line limit set to 1`. Now, for sure it's a multiline text") .lineLimit(2)

truncationMode
Indica el modo de “corte” que debe tener el texto cuando es demasiado largo y no puede ser mostrado completamente. Por defecto tiene el valor .tail
Text("This is a long text with line limit set to 1 and tuncationMode set to middle. I need more text for ensure is a real multiline text, so I repeat all to say before. `This is a long text with line limit set to 1 and tuncationMode set to middle`. Now, for sure it's a multiline text") .lineLimit(1) .truncationMode(.middle)

background
Permite modificar el color de fondo del texto.
Text("Text with background") .background(Color.yellow)
El color afectará al tamaño que ocupe el propio texto que contenga. Si quisiéramos que ocupara todo el ancho (por ejemplo) tendríamos que asignarle el frame
antes de la propiedad background.
Text("Text with background (frame set)") .frame(maxWidth: .infinity) .background(Color.yellow)

foregroundColor
Permite modificar el color del propio texto.
Text("Text with foreground") .foregroundColor(Color.red)

font
Permite modificar la fuente del texto.
Podemos usar una de las fuentes que viene precargada en la SDK o crearnos una con el enumerador .custom
Text("Text with custom font") .font(.custom("AmericanTypewriter", size: 15))

multilineTextAlignment
Indica la alineación del texto cuando es multilínea. Por defecto es .center
Text("This is a long Text with multiline alignment and set to trailing to view the effect") .multilineTextAlignment(.trailing)

lineSpacing
Indica el espaciado entre las líneas en un texto multilínea. Por defecto el valor es 0
.
Text("This is a long text with line spacing and large title for show de line spacing modification") .font(.title) .lineSpacing(30)
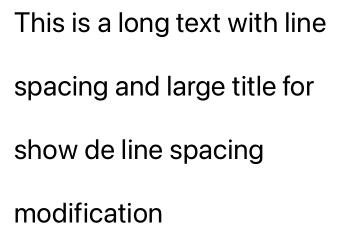
traking
y kerning
Permite modificar el espacio entre los caracteres. La diferencia entre los dos modificadores es que kerning
mantiene las ligaduras del texto mientras de tracking
las rompe. El valor podrá ser positivo (más separación) o negativo (más junto).
Text("kerning: ffi") .font(.custom("AmericanTypewriter", size: 30)) .kerning(20) Text("tracking: ffi") .font(.custom("AmericanTypewriter", size: 30)) .tracking(20)

strikethrough
Tacha el texto.
Text("Text strikethrough") .strikethrough()
Permite modificar el color de la línea que tacha el texto y decidir si se tacha o no a través de un bool.
Text("Text strikethrough red") .strikethrough(true, color: Color.red)

underline
Subraya el texto.
Text("Text underline") .underline()
Permite modificar el color de la línea que subraya el texto y decidir si se subraya o no a través de un bool
.
Text("Text underline red") .underline(true, color: Color.red)

baseline
Indica la separación en la vertical contra la base del texto.
Text("Text baseline") .baselineOffset(30) .background(Color.yellow)

¿Cómo mostrar el texto en HTML?
SwiftUI
aún no incluye ningún componente para mostrar texto HTML por lo que para solucionar este problema tenemos que crearnos nuestro propio componente con la capacidad de pintar texto HTML.
Para ello debemos crearnos un struct que implemente el protocolo UIViewRepresentable
. Este protocolo proporciona la implementación necesaria para que cualquiera pueda ser una vista. En nuestro caso vamos a ‘enmascar’ un UILabel
para que podamos usarlo directamente desde SwiftUI.
import SwiftUI import UIKit struct TextHtml: UIViewRepresentable { let html: String? init(_ html: String?) { self.html = html } func makeUIView(context: UIViewRepresentableContext) -> UILabel { let label = UILabel() DispatchQueue.main.async { if let html = html, let data = html.data(using: .utf8), let attributedString = try? NSAttributedString(data: data, options: [.documentType: NSAttributedString.DocumentType.html], documentAttributes: nil) { label.attributedText = attributedString } } label.numberOfLines = 0 return label } func updateUIView(_ uiView: UILabel, context: UIViewRepresentableContext ) { } }
De esta forma ya tendremos el componente textHTML
disponible para usar en SwiftUI
.
TextHtml("HTML text This work fine.
")
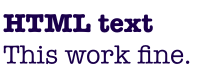
Ejemplo
Puede encontrar este ejemplo en GitHUb aquí bajo el apartado Text.
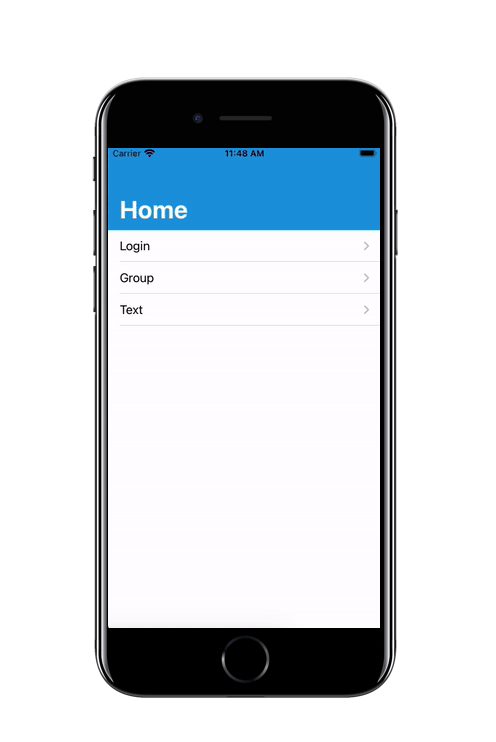
¡La próxima semana seguiremos con más información sobre SwiftUI!
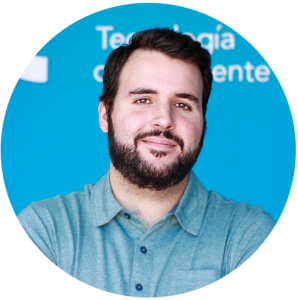
Rafael Fernández,
iOS Tech Lider